728x90
함수의 선언과 표현
오늘 내가 배운 것
- function + 함수 이름 + (param1, param2) { 기본적인 로직 + return;} 의 구조로 이루어진다
- 하나의 함수는 한 가지의 일만 하도록 만들어야 한다.
- 함수의 이름은 동작하는 것 이기 때문에 do-something, 명령어 구조, 동사형태로 작성해야 한다.
- 함수의 이름을 짓기 어렵다면 함수에 정의되어있는 일이 너무 많지 않은지 확인해볼 필요가 있다. (깨끗하게 만들자)
- 자바 스크립트에서 function은 object의 일종이다 그래서 function 이 변수, 파라미터, 함수를 리턴할 수도 있게 된다.
(나중에 object, prototype에서 다시 다룰 예정)
JS에서는 타입이 없다. 그렇기 때문에 함수 자체의 인터페이스를 봤을 때 이 변수가 문자열을 전달해야 하는지 숫자열을 전달해야하는지 명확하지가 않다. Type이 중요한 경우엔 JS로 는 힘들다. (TypeScript도 배워야 한다!!)
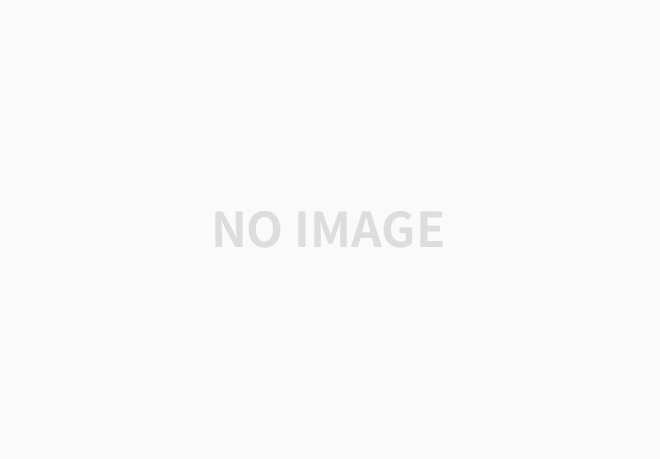
… 을 사용하면 rest parameters(나머지 매개변수) 라고 불리는데 배열형태로 전달되게 된다. 나머지 매개변수는 정해지지 않은 개수의 인수를 배열로 나타낼 수 있게 함
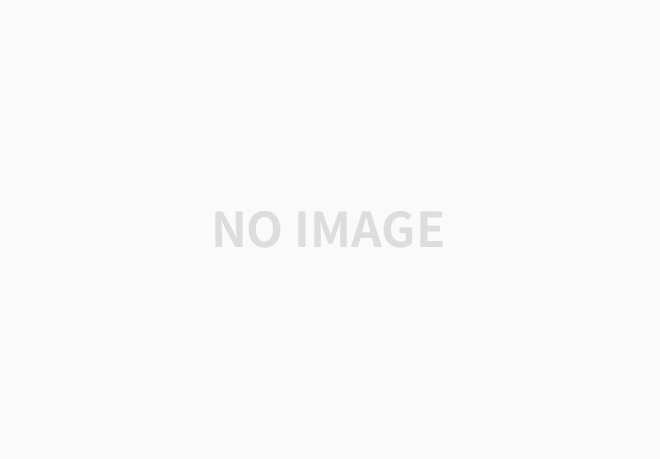
위에 for문을 for-of 를 이용해서 간단하게 출력 가능args에있는 모든 값들이 차례차례arg에출력된다.
결과는 같음.
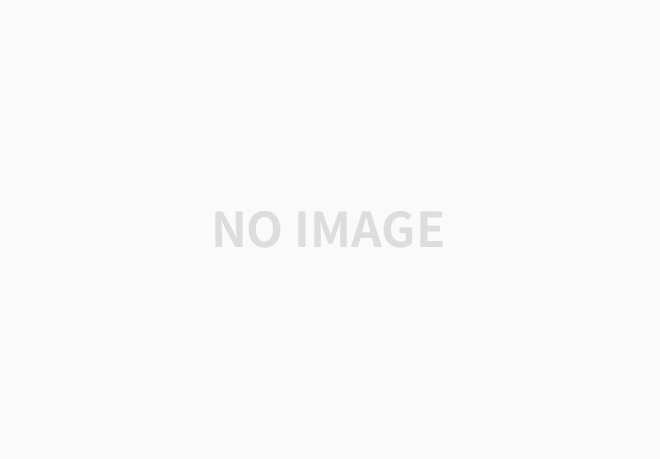
for문 자체를 안 쓰고 배열에서배우는 함수형언어 forEach 를 사용하면 더 간단하게 출력할 수 있다. (배열에서 다시 배울 예정)
자바스크립트에서scope는안에서는 밖이 보이지만 밖에선 안을 볼 수 없다 (유리창 틴팅)
블럭안에서는 블럭밖에 있는 변수를 접근해서 출력이 가능하지만 반대로 블럭 밖에서 블럭안에있는 변수를 접근해서 출력은 불가능하다
동영상 보면서 따라 적어보기
// Function
// - fundamental(기본적인) building block in the program
// - subprogram can be used multiple times (여러번 재사용이 가능)
// - performs a task or calculates a valus.
// 1. Function declaration
// function name(param1, param2) {body.... return;}
// one function === one thing
// naming : doSomething, command, verb
//e.g. createCardAndPoint -> creaeCard, createPoint
//function is object in JS
function printHello(){
console.log('Hello');
}
printHello();
function log(message){
console.log(message)
}
log('Hello!');
log(1234);
// 2. Parameters
//premitive parameters : passed by value
//object parameters : passed by reference
function changeName(obj) {
obj.name = 'coder';
}
const ellie = { name : 'ellie'};
changeName(ellie);
console.log(ellie);
// 3. Default parameters (added in ES6)
function showMessage(message, from) {
console.log(` ${message} by ${from}`);
}
showMessage('Hi!'); //from 이 지정되지 않았다.
// 4. Rest parameters (added in ES6)
function printAll(...args) {
for ( let i = 0; i < args.length; i++) {
console.log(args[i]);
}
for (const arg of args) {
console.log(arg);
} //for of 를 이용해서 간단하게 출력 가능 args 에 있는 모든 값들이 차례차례 arg 에 지정되어 출력된다.
args.forEach((arg) => console.log(arg));
//배열에서 배우는 함수형언어 forEach 를 사용하면 더 간단하게 출력할 수 있다. (배열에서 다시 배울 예정)
}
printAll('dream', 'coding', 'ellie');
// 5. Local scope
let globalMessage = 'global'; // global variable
function printMessage (){
let message = 'hello';
console.log(message); // local variable
console.log(globalMessage);
}
// console.log(message); // error
printMessage();
// 6. Retrun a value
function sum (a, b) {
return a+b;
}
const result = sum(1, 2); //3
console.log(`sum : ${sum (1,2)}`);
// 7. Early return, early exit
// 안좋은 예... 블럭안에서 로직이 많아지면 가독성이 좋지않다.
//if, else 를 쓰는 것 보다 조건이 맞지않을 땐 빨리 리턴을 해서 함수를 종료하고 필요한 로직을 실행하는게 좋다.
function upgradeUser(user) {
if(user.point > 10) {
//long upgrade logic...
}
}
//좋은 예 ...
function upgradeUser(user){
if(user.point <= 10 ){
return;
}
// long upgrade logic...
}
//First-class function
//function are treated like any other variable
//can be assigned as a value to variable. //변수에 할당이 될 수 있고.
//can be passed as an argument to other functions. // function 에 파라미터에 전달이 가능하고
//can be returned by another function // return 값으로도 리턴할 수 있다.
//여기 까지가 위에 배웠던 내용...
반응형
'시작 > TIL(Today I Learned)' 카테고리의 다른 글
[유튜브 '드림코딩'] 자바스크립트(JavaScript ES6) 7강 (1) (0) | 2022.09.29 |
---|---|
[유튜브 '드림코딩'] 자바스크립트(JavaScript ES6) 6강 (2) (0) | 2022.09.28 |
[유튜브 '드림코딩'] 자바스크립트(JavaScript ES6) 5강(2) ~6강 (1) (0) | 2022.09.27 |
[유튜브 '드림코딩'] 자바스크립트(JavaScript ES6) 4강 (2) | 2022.09.24 |
[유튜브 '드림코딩'] 자바스크립트(JavaScript ES6) 3강 (0) | 2022.09.24 |